To install Next.js, you’ll need Node.js version 10.13 or later.
In order to check your current version simply type “node –version” in your terminal (Mac, Linux) or cmd (Windows). If you have an older version, you can get the latest one from here.
Using Create Next App
The easiest way to create a Next.js application is by using create-next-app. It is actually the same thing as create-react-app except it creates a Next app for you instead of React app.
To start, type the command shown below in your terminal or cmd.
npx create-next-app
# or
yarn create next-app
To use TypeScript you’ll have to provide the “–ts” or “–typescript” flag.
npx create-next-app --ts
# or
yarn create next-app --typescript
If everything went fine, you should see a piece of success information in your terminal. To start the development use one of these commands.
npm run dev
# or
yarn dev
To see how it looks like, let’s follow suggestions from the terminal and head to localhost:8000
And that’s it. We have created a very basic Next.js application using create-next-app. To learn more about create-next-app and how to create production build please visit the documentation here.
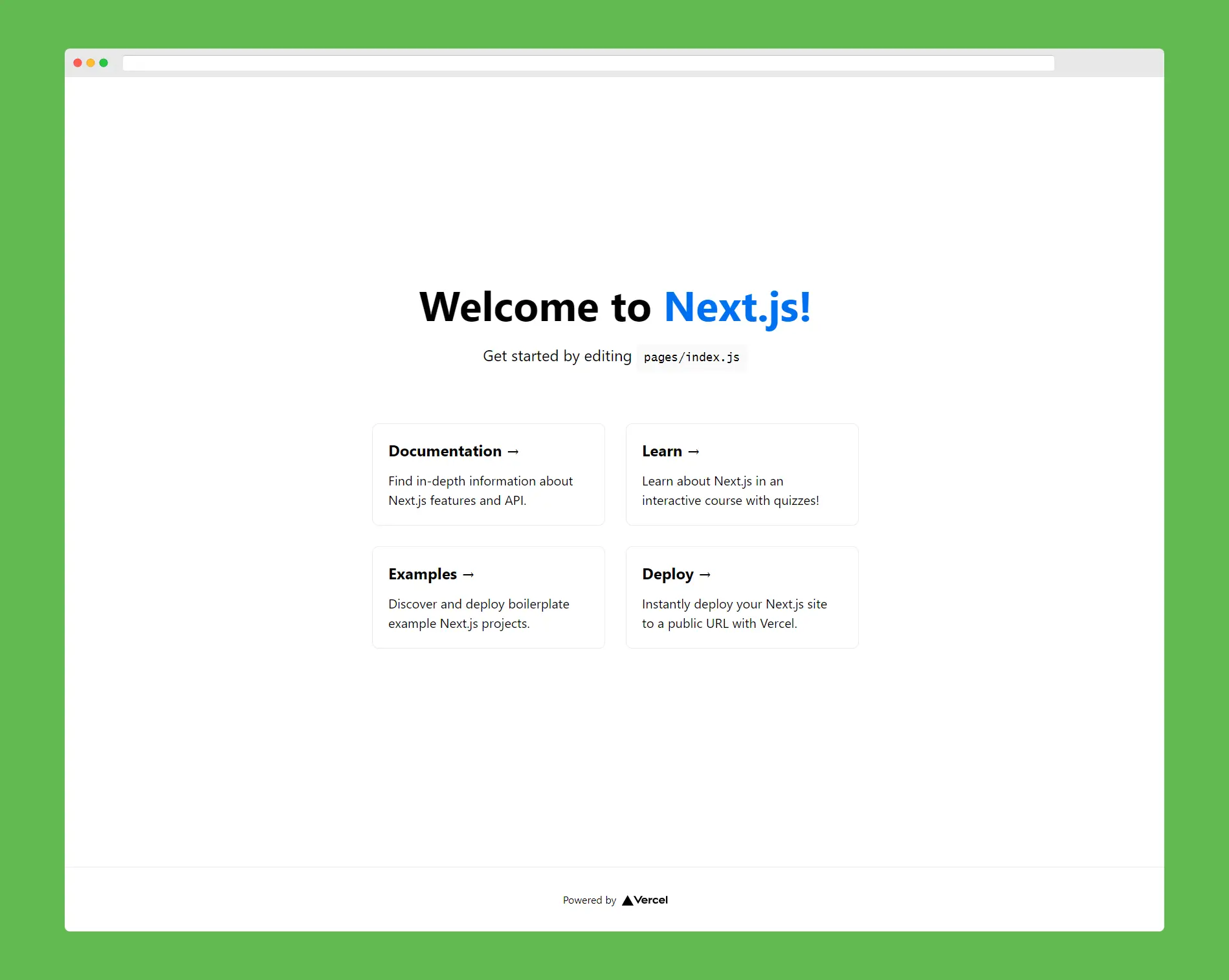
Manually create Next.js project
You don’t always have to use create-next-app while initializing your project if there are any reasons you want to avoid using it or you simply want to create the Next app from scratch, you can do it in just a few simple steps.
1. Create a new empty directory and initialize Node project with npm init.
# Create empty directory
mkdir teacode-rocks
# Move to /teacode-rocks directory
cd teacode-rocks
# Initialize Node project
npm init -y
2. Install next, react and react-dom.
npm install next react react-dom
3. Add scripts to your package.json file.
"scripts": {
"dev": "next",
"build": "next build",
"start": "next start"
}
4. Add /pages directory and create _app.js file inside it.
function MyApp({ Component, pageProps }) {
return <Component {...pageProps} />;
}
export default MyApp;
5. Create an index.js inside it.
import Head from 'next/head'
export default function Home() {
<div>
<Head>
<title>Teacode Rocks!</title>
</Head>
<main>
<h1>This is my manually set-up Next.js project!</h1>
</main>
</div>
}
npm run dev
In order to find out how to customize your manual set-up Next.js app and use advanced features visit Next.js documentation here.
Now you are ready to go with your very default Next.js project!